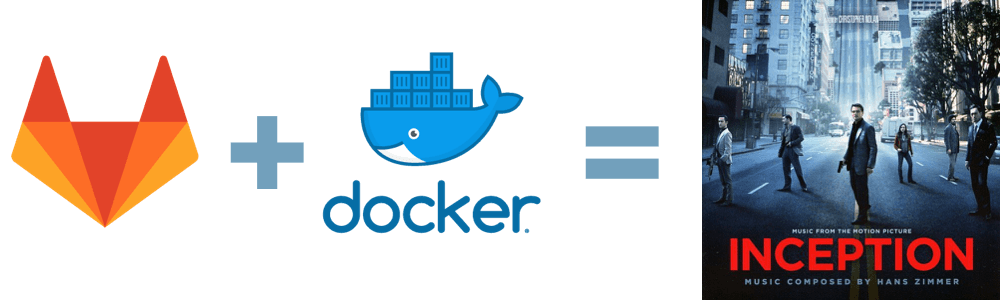
GitLab Docker in Docker with Postgres
NOTE: If you just need to build docker in a GitLab Pipeline, you should use Kaniko instead of DIND.
I’m embarrassed this took me longer than expected, but here is a .gitlab-ci.yml
that will fire up Docker in Docker (so you can build and run containers) and run Postgres to use in your contract tests.
image: docker:stable
stages:
- build
- test
# When using dind, it's wise to use the overlayfs driver for
# improved performance.
variables:
DOCKER_DRIVER: overlay2
services:
- docker:dind
# These are here just for info purposes (login is needed for GitLab registry)
before_script:
- docker version
- docker info
- docker login registry.gitlab.com -u $GITLAB_USERNAME -p $GITLAB_TOKEN
# This is where we build our image, and tag it with the git commit hash, then push to GitLab registry
build:
stage: build
script:
- docker build -t $GITLAB_IMAGE_NAME:$CI_COMMIT_SHA -t $GITLAB_IMAGE_NAME:latest .
- docker run --rm $GITLAB_IMAGE_NAME:$CI_COMMIT_SHA npm run lint
- docker push $GITLAB_IMAGE_NAME:$CI_COMMIT_SHA
- docker push $GITLAB_IMAGE_NAME:latest
# Run contract tests
contract:
variables:
DATABASE_URL: postgres://postgres@postgres/postgres
stage: test
script:
- docker run -d -e POSTGRES_DB -e POSTGRES_USER -e POSTGRES_PASSWORD --name postgres postgres:9.6
- docker pull $GITLAB_IMAGE_NAME:$CI_COMMIT_SHA
- docker run --rm -e DATABASE_URL --link postgres $GITLAB_IMAGE_NAME:$CI_COMMIT_SHA npm run contract
In this config, I have some environment variables configured in the gitlab.com UI.
- GITLAB_IMAGE_NAME: Something like
registry.gitlab.com/project/repo
- GITLAB_USERNAME: Your username on GitLab
- GITLAB_TOKEN: A token you can generate at https://docs.gitlab.com/ee/user/profile/personal_access_tokens.html
You’ll notice, after I build, I run npm run lint
in the container, then after PG is started, we run npm run contract
. These are scripts declared in your package.json
file.
For the curious, my Dockerfile is
FROM node:10-alpine
RUN apk update && \
apk upgrade && \
mkdir /src
# I used pm2 for process management
RUN npm i -g pm2
# If you use any private repos, then you'll need this
#ARG NPM_TOKEN
#RUN echo "//registry.npmjs.org/:_authToken=${NPM_TOKEN}" > src/.npmrc
COPY package.json package-lock.json src/
ARG NODE_ENV
RUN cd src/ && npm install
COPY . src/
WORKDIR src/
EXPOSE 3000
CMD [ "pm2-runtime", "start", "process.yml" ]