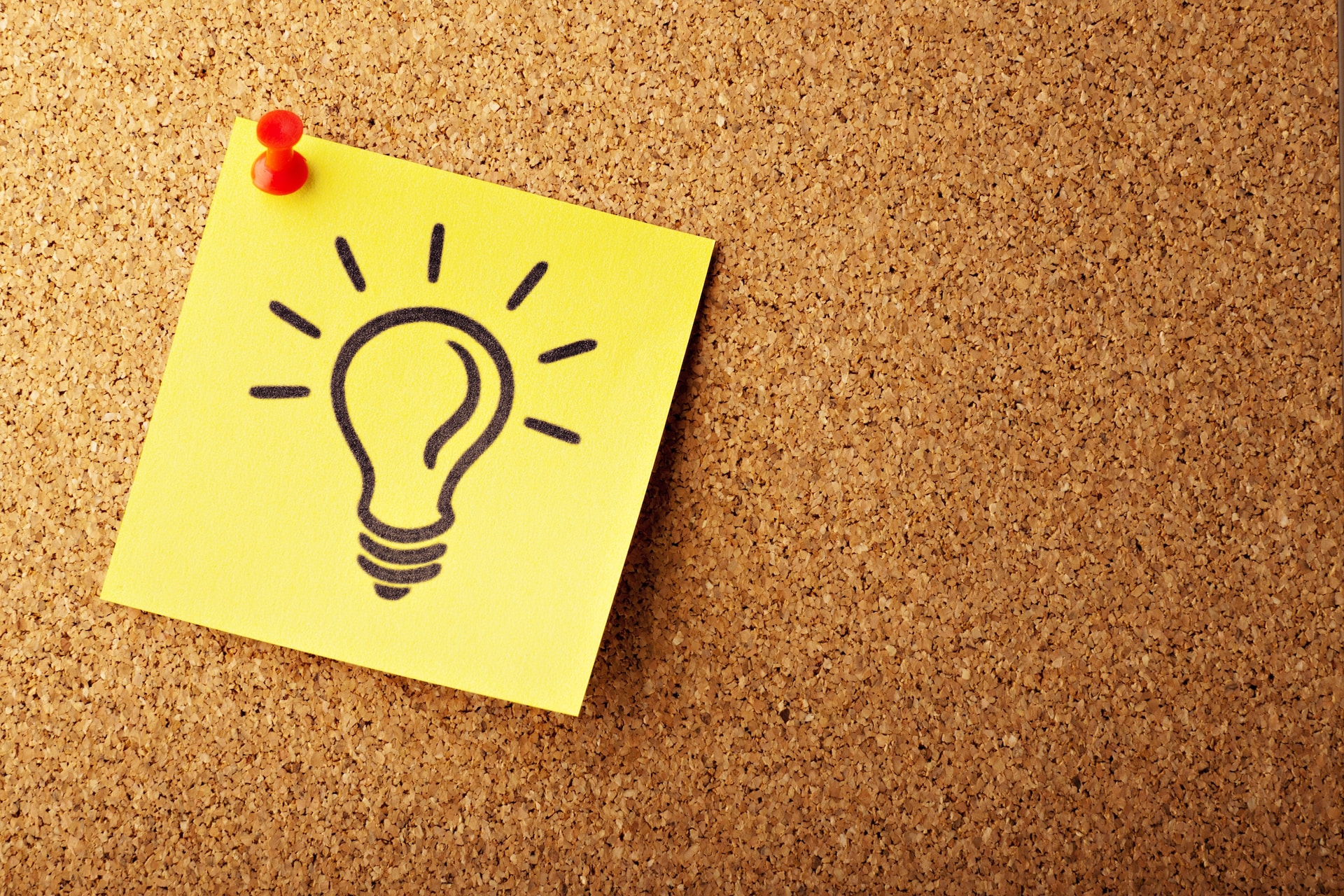
JavaScript Casting Tricks
Hold on Cowboy
This blog post is pretty old. Be careful with the information you find in here. The Times They Are A-Changin'
Here I want to go through some casting tricks that can be used for better data manipulation.
Booleans
Build in Boolean()
The easiest way is to use the builtin Boolean()
method.
Boolean('true'); // true
Boolean(1); // true
Boolean('false'); // true !!!!GOTCHA!!!!!
Boolean(0); // false
// Careful
Boolean([]); // true
Double Negative
This will cast anything as either true
or false
, there are of course a few quirks that may not be obvious.
// FALSE
!!false;
!!null;
!!undefined;
!!0;
!!'';
// TRUE
!!true;
!!'true';
!!1;
!!-1;
!!{};
!!function(){};
// GOTCHA!!! ... TRUE
!!'false';
JSON.parse
This will parse the text 'true'
to the boolean true
// TRUE
JSON.parse('true');
// FALSE
JSON.parse('false');
// If using a variable, you'll want a fallback
JSON.parse(undefined || 'true');
JSON.parse('' || 'true');
Numbers
Check for a decimal using modulo
5.5 % 1 != 0; // true
5 % 1 != 0; // false
Reference: http://stackoverflow.com/a/2304062/179335
Strings
Built in tools toString() and String()
var hello = String('hello')
10.0.toString();
Casting an Array as a String
Strings are of course mostly just an array of characters so arrays and strings have a lot in common. You can cast an array to a string simply by joining them with nothing.
var hello = ['h', 'e', 'l', 'l', 'o'];
hello.join(''); // Yields "hello"
Convert from an Array to a String
Strings can be converted to an array easily using split
. Here’s how.
var hello = "hello";
hello.split(''); // ['h', 'e', 'l', 'l', 'o'];
Arguments
In JavaScript the arguments object in a function is array like, but to convert it to an actually array you can just use a simple trick.
Array.prototype.slice.call(arguments);
Or more simply if Array generics are available (like in node.js).
Array.slice(arguments);