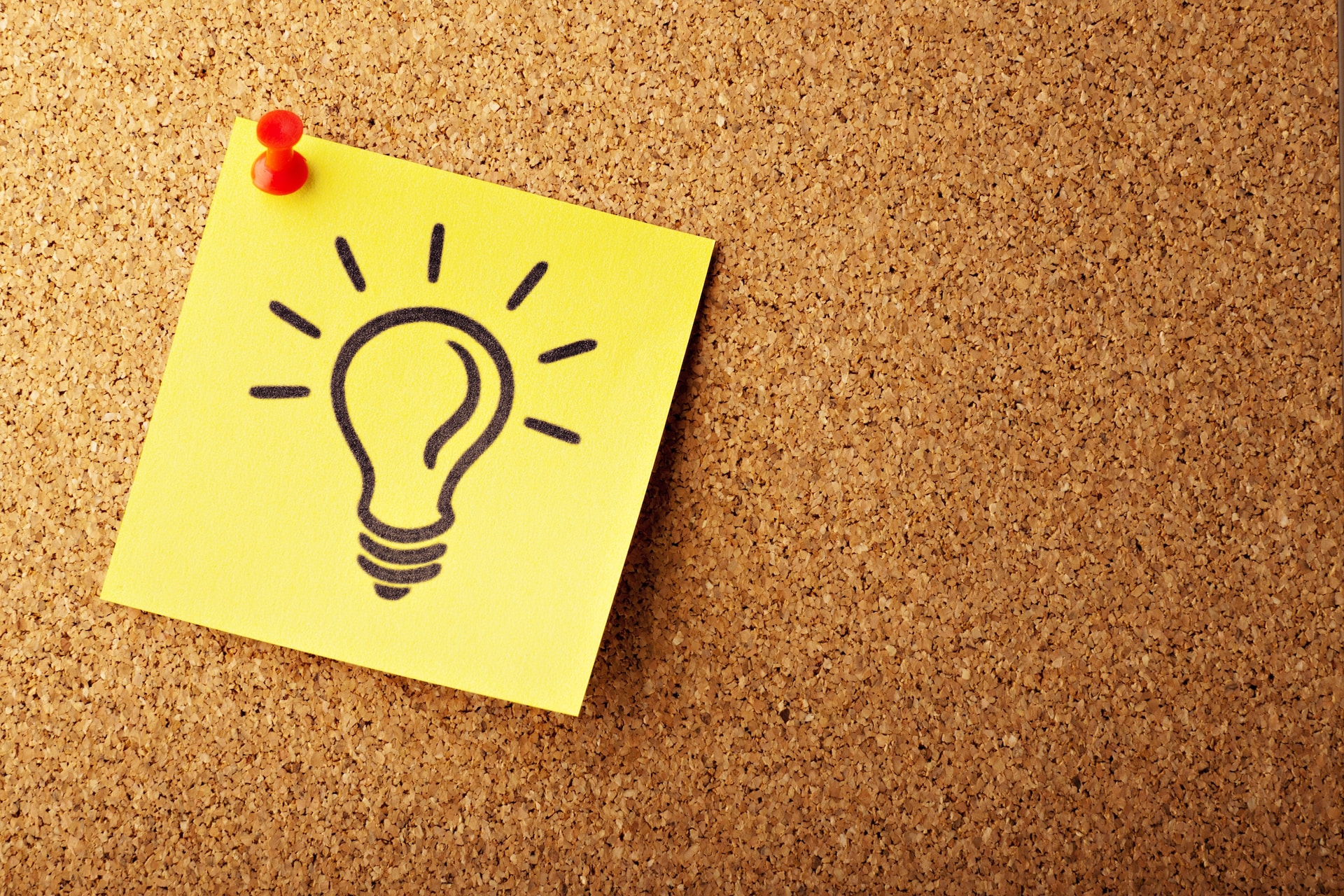
PHP SimpleXmlElement values into an array
Hold on Cowboy
This blog post is pretty old. Be careful with the information you find in here. The Times They Are A-Changin'
I have an XML string I was trying to parse for some values. The following XML was stored in a variable
<?xml version="1.0" encoding="UTF-8"?>
<ns0:Item xmlns:ns0="http://www.example.com/schemas/Subscribers/Customer_Item.xsd">
<ns0:Style>C3399</ns0:Style>
<ns0:Description>Lion's Paw Shell Pendant</ns0:Description>
<ns0:Status>Active</ns0:Status>
<ns0:Quantity_Available>1</ns0:Quantity_Available>
<ns0:Wholesale_Price>20.59</ns0:Wholesale_Price>
</ns0:Item>
I used this to get to the values into an array.
<php>
$xmlObj = new SimpleXMLElement($xml);
$item = $xmlObj->children('ns0', TRUE);
$data = array();
$data['Style'] = $item->Style;
$data['Status'] = $item->Status;
$data['Qty_Avail'] = $item->Quantity_Available;
$data['Price'] = $item->Wholesale_Price;
</php>
But when I did a
<php>
Array
(
[Style] => SimpleXMLElement Object
(
[0] => C3399
)
[Status] => SimpleXMLElement Object
(
[0] => Active
)
[Qty_Avail] => SimpleXMLElement Object
(
[0] => 1
)
[Price] => SimpleXMLElement Object
(
[0] => 20.59
)
)
</php>
But for testing if I just did aC3399
Then I ran across this little advice “You should cast elements to a float (or even string) if you plan on using them…” That made all the difference. So now my code has the (string)
casting.
<php>
$xmlObj = new SimpleXMLElement($xml);
$item = $xmlObj->children('ns0', TRUE);
$data = array();
$data['Style'] = (string)$item->Style;
$data['Status'] = (string)$item->Status;
$data['Qty_Avail'] = (string)$item->Quantity_Available;
$data['Price'] = (string)$item->Wholesale_Price;
</php>
Now I get a nice array, like I was expecting.
<php>
Array
(
[Style] => C3399
[Status] => Active
[Qty_Avail] => 1
[Price] => 20.59
)
</php>
Resources: http://www.php.net/manual/en/class.simplexmlelement.php